Interfacing an Ultrasonic Sensor (HC-SR04) with Arduino
- Varghese Benny
- Oct 23, 2024
- 2 min read
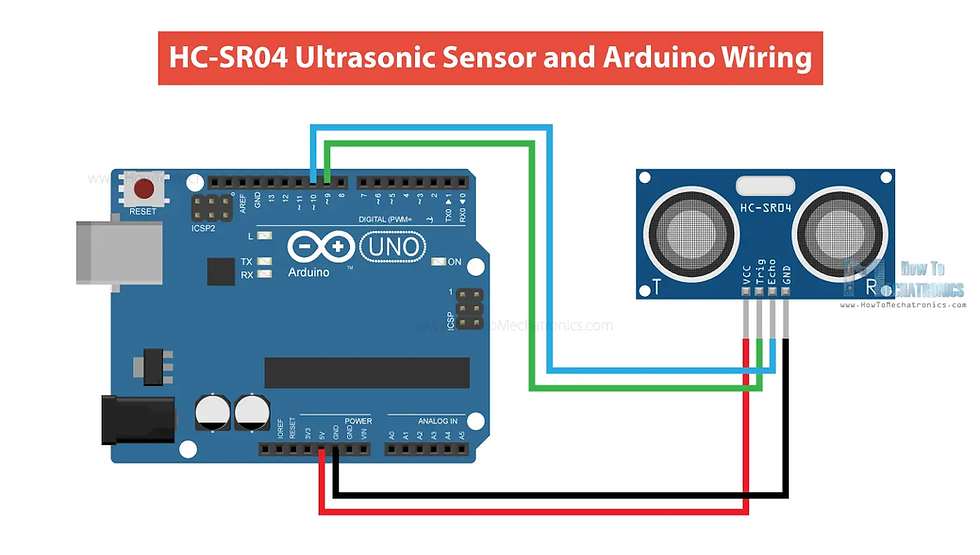
What is an Ultrasonic Sensor?
An ultrasonic sensor measures distance by emitting ultrasonic waves and calculating the time it takes for the sound waves to bounce back after hitting an object. It typically has two main components: a transmitter that emits the sound waves and a **receiver** that detects the reflected waves. The sensor uses the speed of sound (approximately 343 m/s in air) to calculate the distance to an object.
Ultrasonic sensors are commonly used in robotics for obstacle detection, in automation systems for distance measurement, and in parking assistance systems for vehicles.
Components:
- Arduino (any model, like Uno)
- Ultrasonic Sensor (HC-SR04)
- Jumper wires
- Breadboard (optional)
HC-SR04 Pin Description:
- VCC → 5V power supply
- GND → Ground
- Trig→ Trigger pin (to send ultrasonic pulses)
- Echo → Echo pin (to receive the reflected pulse)
#### Wiring the Ultrasonic Sensor to Arduino:
1. VCC→ 5V pin on Arduino
2. GND → GND pin on Arduino
3. Trig → Digital Pin 9 on Arduino
4. Echo → Digital Pin 10 on Arduino
Code Example:
const int trigPin = 9; // Pin connected to the Trig pin of the sensor
const int echoPin = 10; // Pin connected to the Echo pin of the sensor
long duration; // Variable to store the duration of the pulse
int distance; // Variable to store the calculated distance
void setup() {
pinMode(trigPin, OUTPUT); // Set the Trig pin as output
pinMode(echoPin, INPUT); // Set the Echo pin as input
Serial.begin(9600); // Initialize serial communication for debugging
}
void loop() {
// Clear the trigger pin
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Set the trigger pin HIGH for 10 microseconds to send the pulse
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Read the echo pin and calculate the time it took for the pulse to return
duration = pulseIn(echoPin, HIGH);
// Calculate the distance (Speed of sound = 34300 cm/s)
distance = duration * 0.034 / 2; // Divide by 2 to account for the round trip of the pulse
// Print the distance to the Serial Monitor
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(500); // Small delay for stability
}
How the Code Works:
1. Triggering the Pulse: The Arduino sends a 10-microsecond pulse through the Trig pin to start the measurement.
2. Reading the Echo: The **Echo** pin listens for the reflected pulse. The time between the pulse emission and its return is captured using the `pulseIn()` function.
3. Distance Calculation: The time taken for the pulse to travel to the object and back is divided by 2 and multiplied by 0.034 (speed of sound in cm/µs) to calculate the distance.
Testing:
1. Upload the code to your Arduino.
2. Open the Serial Monitor to observe the distance measurements (in centimeters). As you move objects closer or farther from the sensor, the distance values will change accordingly.
Comments